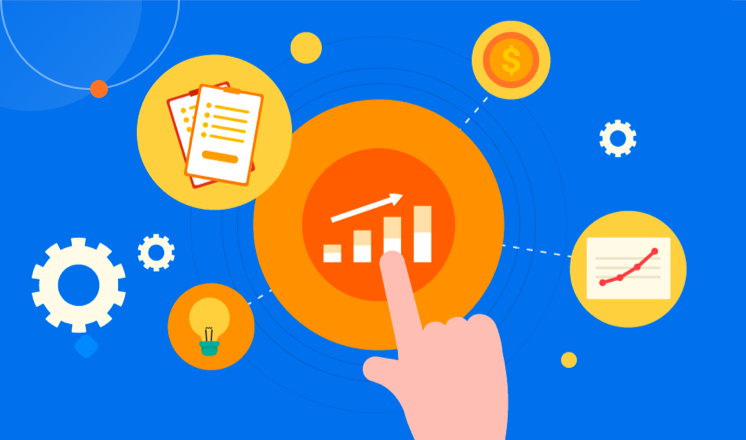
Puppeteer is a powerful tool for automating web tasks, such as web scraping, form submission, and UI testing. However, when it comes to web scraping, using proxies is essential to avoid getting blocked by websites. In this article, we will explore how to set up and use proxies with Puppeteer, specifically focusing on the proxy-chain library for rotating proxies.
### What is Puppeteer Proxy?
Puppeteer Proxy refers to the ability to route Puppeteer's web requests through a proxy server. This allows you to hide your IP address and make requests appear to be coming from a different location, which is crucial for web scraping and data collection.
### Setting up Proxy with Puppeteer
To set up a proxy with Puppeteer, you can use the proxy-chain library, which provides a simple and efficient way to route Puppeteer's requests through a proxy server. Here's a step-by-step guide to setting up a proxy with Puppeteer using proxy-chain:
1. Install proxy-chain library using npm:
```bash
npm install proxy-chain
```
2. Require the proxy-chain library in your Puppeteer script:
```javascript
const { addProxy, getProxy } = require('proxy-chain');
const puppeteer = require('puppeteer');
```
3. Set up the proxy server and add it to Puppeteer's launch options:
```javascript
const proxyUrl = 'http://your-proxy-server.com:8000';
const browser = await puppeteer.launch({
args: [`--proxy-server=${proxyUrl}`],
});
```
### Using Rotating Proxies with Puppeteer
Rotating proxies are essential for web scraping at scale, as they help distribute requests across multiple IP addresses, reducing the chance of getting blocked. With the proxy-chain library, you can easily implement rotating proxies in your Puppeteer scripts. Here's how you can achieve this:
1. Create a pool of rotating proxies using the proxy-chain library:
```javascript
const proxyUrls = ['http://proxy1.com:8000', 'http://proxy2.com:8000', 'http://proxy3.com:8000'];
const proxyServers = [];
for (const url of proxyUrls) {
const proxy = await addProxy({ proxyUrl: url });
proxyServers.push(proxy);
}
```
2. Use the rotating proxies in your Puppeteer script:
```javascript
const browser = await puppeteer.launch({
args: [`--proxy-server=${await getProxy()}`],
});
```
### Conclusion
In conclusion, using proxies with Puppeteer is crucial for web scraping and automation tasks. The proxy-chain library provides a convenient way to set up and use proxies, including rotating proxies, with Puppeteer. By leveraging proxies, you can effectively scrape data from websites without the risk of being blocked. Start implementing proxies in your Puppeteer scripts today and take your web automation to the next level!