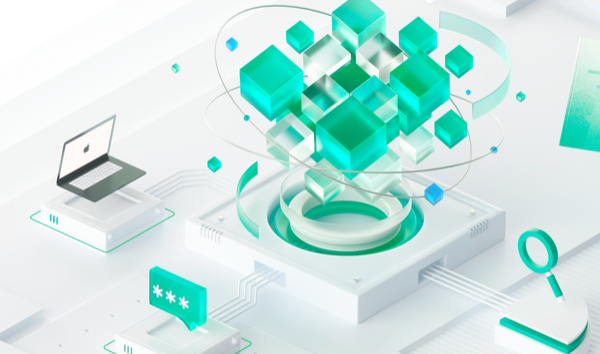
When it comes to web scraping, using a rotating proxy with Selenium in Python can be incredibly useful. In this article, we will explore the concept of rotating proxies and how to integrate them with Selenium for efficient web scraping.
What is a rotating proxy?
A rotating proxy is a type of proxy server that automatically switches between a large number of IP addresses from a proxy pool. This rotation of IP addresses helps to avoid detection and IP bans when scraping data from websites.
Using rotating proxy with Selenium in Python
To use rotating proxy with Selenium in Python, you can leverage libraries such as requests, BeautifulSoup, and the ProxyBroker library. ProxyBroker is a powerful Python library that enables you to find and manage proxies for web scraping tasks. By integrating ProxyBroker with Selenium, you can automate the process of rotating proxies while scraping data from websites.
Here's a simple example of using rotating proxy with Selenium in Python:
```python
from selenium import webdriver
from proxybroker import Broker
# Find a rotating proxy using ProxyBroker
def find_rotating_proxy():
broker = Broker()
proxies = broker.find(types=['HTTP', 'HTTPS'], limit=1)
return next(proxies)
# Set up Selenium with rotating proxy
proxy = find_rotating_proxy()
options = webdriver.ChromeOptions()
options.add_argument('--proxy-server=%s' % proxy.host)
driver = webdriver.Chrome(chrome_options=options)
# Now you can use Selenium to scrape data with rotating proxy
# ...
```
By using the above approach, you can ensure that your web scraping activities are not easily detected and blocked by websites.
Benefits of using rotating proxy with Selenium
- Avoid IP bans: Rotating proxies help to avoid IP bans by constantly changing the IP address used for web scraping.
- Increased anonymity: Rotating proxies enhance anonymity by masking the true IP address of the scraper.
- Scalability: With a large pool of rotating proxies, you can scale your web scraping operations without being limited by IP restrictions.
Conclusion
In conclusion, using rotating proxy with Selenium in Python can greatly improve the efficiency and success rate of web scraping activities. By leveraging the power of rotating proxies, you can scrape data from websites without the fear of being blocked or detected. Remember to use rotating proxies responsibly and respect the terms of service of the websites you are scraping.