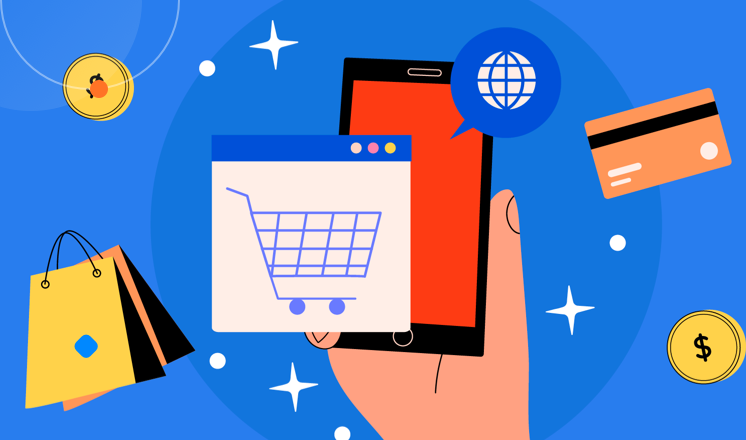
When it comes to web scraping with Selenium in Python, using rotating proxies can be a game changer. Rotating proxies allow you to make multiple requests from different IP addresses, thus preventing your IP from getting blocked by target websites. In this article, we will explore how to integrate rotating proxies with Selenium to enhance the efficiency and reliability of your web scraping projects.
First, let's understand the concept of rotating proxies. A rotating proxy is a type of proxy server that automatically changes the IP address for each request. This rotation of IP addresses helps to distribute the web scraping traffic across multiple IP addresses, making it more difficult for websites to detect and block the scraping activity.
To implement rotating proxies with Selenium in Python, you can leverage third-party libraries such as 'selenium-requests' or 'selenium-wire' that provide built-in support for proxy rotation. These libraries allow you to seamlessly integrate rotating proxies with your Selenium web driver, enabling you to switch IP addresses for each request.
Here's a basic example of using rotating proxies with Selenium in Python:
```
from selenium import webdriver
from selenium.webdriver.chrome.options import Options
proxy_list = ['proxy1:port', 'proxy2:port', 'proxy3:port']
options = Options()
options.add_argument('--proxy-server=http://{}'.format(proxy_list[0]))
driver = webdriver.Chrome(options=options)
driver.get('https://www.example.com')
```
In this example, we create a list of rotating proxies and use Selenium's ChromeOptions to set the proxy for the web driver. By cycling through the proxy list for each request, we can achieve IP rotation and avoid detection by target websites.
It's important to note that while rotating proxies can help mitigate IP bans and improve anonymity, they should be used responsibly and in compliance with the terms of service of the target websites. Additionally, using free rotating proxies may come with limitations and reliability issues, so consider investing in premium rotating proxy services for more stable and efficient web scraping operations.
In conclusion, implementing rotating proxies with Selenium in Python can significantly enhance the effectiveness of web scraping projects by enabling IP rotation and preventing IP bans. By leveraging the power of rotating proxies, you can gather data more efficiently and reliably while minimizing the risk of getting blocked by target websites.